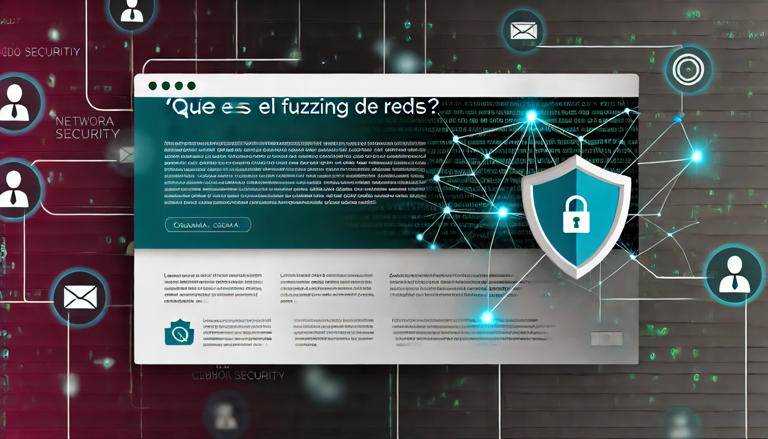
What Is Network Fuzzing?
Network fuzzing is a technique for automatically detecting software errors. It involves trying random correct and incorrect inputs to trigger software vulnerabilities.
As networks get more complicated and connected, the chances of cyber security threats increase. As per Forbes Advisor, There were 2,365 cyberattacks in 2023 with 343,338,964 victims. In such cases, network fuzzing steps in to tackle this risk by finding vulnerabilitiesbefore they’re misused by attackers. This gives you a heads-up to fix things before they cause serious problems.
Importance Of Network Fuzzing In Network Security
Network fuzzing is like a fire drill. It identifies and rectifies security vulnerabilities before they can be exploited, giving a proactive measure to shield against security breaches. Network fuzzing helps to keep your network safe and secure. It is an essential tool to fight against cyber security threats. Plus–it can reduce the risk of security breaches and provide an external security layer to infrastructure. Undoubtedly, it is an essential part of any network security strategy.
General Principles Of Effective Fuzzing Techniques
Fuzzing testing involves giving valid and invalid inputs in software to get responses. Its objective is to target vulnerabilities and weaknesses in software that might lead to any security breach.
So, here are the basic concepts of fuzzy testing:
- Input fields: Fuzzing starts with providing invalid and valid inputs to test the software. Fuzz testing can use manual or automated tools for this purpose.
- Mutation: Fuzzing involves altering input data to produce new test cases. The format, length, or content of the data can be altered to achieve mutation.
- Automation: Fuzzing involves automated processes, running software with large amounts of test cases to identify security vulnerabilities.
- Monitoring: Fuzzing also monitors the unexpected behaviors during fuzzing like crashes, hangs or memory leaks.
Best Practices To Conduct Fuzz Testing
Fuzz testing involves several steps, such as:
Common Pitfalls To Avoid
An introduction to Scapy and its relevance in network security
Python-based module “Scapy ‘’ is intended for network communication and packet manipulation. Its extensive feature set makes it possible to create personalized network traffic, which makes it the perfect tool for fuzzing. Because of Scapy’s versatility, users can precisely control the contents of packets they create, making it possible to enable target fuzzing of specific protocols.
Protocol Fuzzing Using Scapy
Protocol fuzzing using Scapy is a Python library for network packet generation and manipulation. To get started with Scapy, you first need to install it using pip:
$ pip install scapy
Once installed, you can use Scapy to craft packets. For example, to create a simple ICMP packet, you can use the following code:
from scapy.all import *
ip = IP(dst="192.168.1.1")
icmp = ICMP()
packet = ip/icmp
response = sr1(packet, timeout=2, verbose=0)
if response:
print(f"Source IP: {response[IP].src}")
print(f"Source Port: {response[IP].sport}")
else:
print("No response received")
To perform protocol fuzzing, you can modify the packet by changing its fields or adding new ones. For example, to fuzz the ICMP type field, you can use the following code:
from scapy.all import *
ip = IP(dst="192.168.1.1")
icmp = ICMP(type=RandShort()) # Random ICMP type value
packet = ip/icmp
response = sr1(packet, timeout=2, verbose=0)
if response:
print(f"Source IP: {response[IP].src}")
print(f"Source Port: {response[IP].sport}")
else:
print("No response received")
In this example, we changed the ICMP type field to a random value between 0 and 255 using the RandShort()
function. By sending a large number of packets with different values, you can test the robustness of the network protocol and identify any vulnerabilities.
Fuzzing Concepts: A Lousy Way To Do Fuzzing And The Correct One To Do It
In network security, fuzzing is a method used to figure out how reliable network protocols are. It involves bombarding a system with a significant amount of incorrect, unexpected, or random data packets in an attempt to cause unexpected behavior, including crashes or hangs. Finding software, service, or device vulnerabilities before malevolent actors can take advantage of them is the aim of fuzzing.
Fuzzing can be done in two ways: the incorrect way and the correct way. Sending arbitrary data packets to a system with no structure or goal is a poor method. It’s doubtful that this method will find any vulnerabilities, and it might even lead to false positives. Understanding the network protocol under test and creating packets that take advantage of potential flaws are essential components of proper fuzzing techniques. This method has a higher chance of identifying weaknesses and offering useful information.
Examples Of Simple Fuzzing Scripts And How To Interpret Their Outputs:
Here is an example of a simple fuzzing script using Scapy.
from scapy.all import *
# Define the target IP address and port number
target_ip = "192.168.1.1"
target_port = 80
# Define the number of packets to send
num_packets = 100
# Define the fuzzing function
def fuzz(packet):
# Modify the payload of the packet with random data
payload = "A" * (packet.len - 28)
return IP(dst=target_ip)/TCP(sport=RandShort(), dport=target_port)/payload
# Send the fuzzed packets
for i in range(num_packets):
packet = IP(dst=target_ip)/TCP(sport=RandShort(), dport=target_port)
fuzzed_packet = fuzz(packet)
send(fuzzed_packet)
# Analyze the results
answered_packets = sr1(IP(dst=target_ip)/TCP(dport=target_port), timeout=5, verbose=0)
if answered_packets:
print(f"Received response from {answered_packets[IP].src}:{answered_packets[TCP].sport}")
else:
print("No response received")
This script sends a number of TCP packets to a target IP address and port with a randomized payload. The sr1()
function is then used to receive a response from the target, if any. If a response is received, the source IP address and port number are printed.
To interpret the output, you can look for any unexpected behavior in the response, such as crashes or hangs. If no response is received, it may indicate that the target is not responding to the fuzzed packets, or that the packets are not being sent correctly.
To conclude, best practices for conducting fuzzing tests include defining the scope, selecting appropriate fuzzing tools, generating a large number of test cases, monitoring the test for signs of unexpected behaviour, analyzing the results, and reporting any vulnerabilities found. Common pitfalls to avoid include lack of scope definition, using the wrong fuzzing tool, poor test case generation, insufficient monitoring, inadequate analysis, and poor reporting.